Tri-Morse-Coder
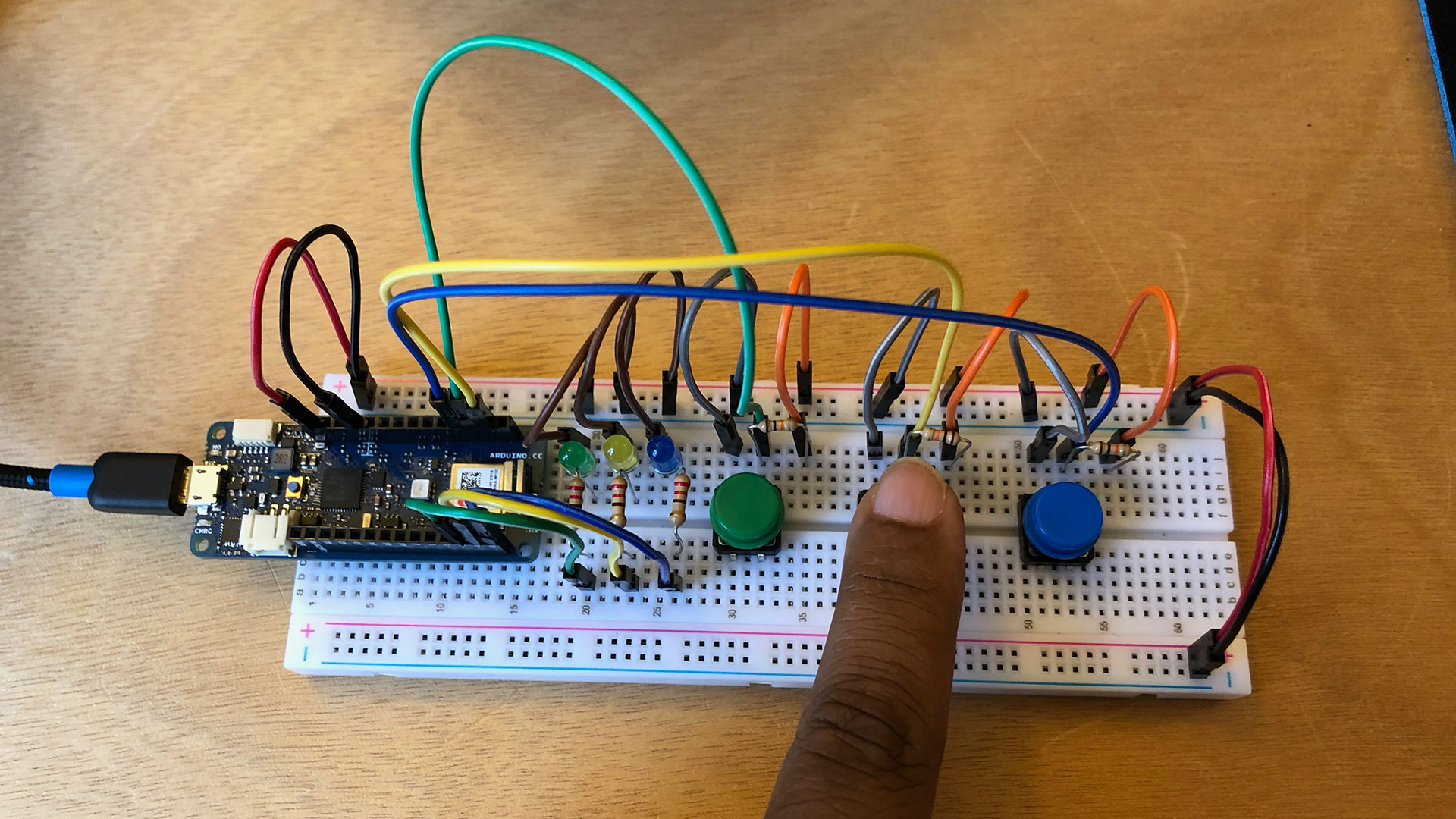
Description:
My goal is to create a modern telegraph machine that replaces the subjective interpretation of “dots”, “dashes” and “spaces” with independent objective buttons to hopefully improve functionality, speed and online delivery.
Goals:
- Send three independent button signals to an online web page.
- Interpret said signals as either “dot”, “dash” or “space”.
- Display said signals online via text and graphically.
Lessons Learned:
- ALWAYS clear sketch code on Arduino with example code “Bare Minimum” before uploaded new code!
- ALWAYS confirm IOT online connection via the monitor sidebar BEFORE clicking on “GO TO IOT CLOUD” to test the dashboard!
Sample Code:
/* Sketch generated by the Arduino IoT Cloud Thing "MorseTriCode" https://create.arduino.cc/cloud/things/0929142f-09e3-4511-9c57-780b3556dc7f Arduino IoT Cloud Properties description. The following variables are automatically generated and updated when changes are made to the Thing properties String telegraphTape; bool dash_yellow; bool dot_green; bool space_blue; Properties which are marked as READ/WRITE in the Cloud Thing will also have functions which are called when their values are changed from the Dashboard. These functions are generated with the Thing and added at the end of this sketch. */ #include "thingProperties.h" // CONSTS // const int butPinBlue = 9; const int butPinYellow = 7; const int butPinGreen = 8; // const int ledPinBlue = 5; const int ledPinYellow = 4; const int ledPinGreen = 3; // VARS // int butStateBlue = 0; int butStateYellow = 0; int butStateGreen = 0; bool lightOnBlue = false; bool lightOnYellow = false; bool lightOnGreen = false; // CUSTOM TIMERS long lastMesssageTime = 0; long messagedDelay = 50; String lastMessageTimeStr = ""; void setup() { // Defined in thingProperties.h initProperties(); // Initialize serial and wait for port to open: Serial.begin(9600); // This delay gives the chance to wait for a Serial Monitor without blocking if none is found delay(1500); // Connect to Arduino IoT Cloud ArduinoCloud.begin(ArduinoIoTPreferredConnection); /* The following function allows you to obtain more information related to the state of network and IoT Cloud connection and errors the higher number the more granular information you’ll get. The default is 0 (only errors). Maximum is 4 */ setDebugMessageLevel(2); ArduinoCloud.printDebugInfo(); // initialise BUTTONS as INPUT pinMode(butPinGreen, INPUT); pinMode(butPinYellow, INPUT); pinMode(butPinBlue, INPUT); // initialize LED's as OUTPUT pinMode(ledPinGreen, OUTPUT); pinMode(ledPinYellow, OUTPUT); pinMode(ledPinBlue, OUTPUT); } void loop() { ArduinoCloud.update(); // Your code here // // Serial.println("looping"); // button and messenger widget Delay if ( (millis() - lastMesssageTime) > messagedDelay) { Serial.println("Custom Update Loop"); lastMessageTimeStr = String(lastMesssageTime); // telegraphTape = lastMessageTimeStr; butStateBlue = digitalRead(butPinBlue); butStateYellow = digitalRead(butPinYellow); butStateGreen = digitalRead(butPinGreen); if (butStateBlue == LOW) { if (lightOnBlue == false){ // turn LED on: digitalWrite(ledPinBlue, HIGH); lightOnBlue = true; telegraphTape = "Space - " + lastMessageTimeStr; space_blue = true; } } else { // turn LED off: digitalWrite(ledPinBlue, LOW); lightOnBlue = false; space_blue = false; } if (butStateYellow == LOW) { if (lightOnYellow == false) { // turn LED on: digitalWrite(ledPinYellow, HIGH); lightOnYellow = true; telegraphTape = "Dash - " + lastMessageTimeStr; dash_yellow = true; } } else { // turn LED off: digitalWrite(ledPinYellow, LOW); lightOnYellow = false; dash_yellow = false; } if (butStateGreen == LOW) { if (lightOnGreen == false) { // turn LED on: digitalWrite(ledPinGreen, HIGH); lightOnGreen = true; telegraphTape = "Dot - " + lastMessageTimeStr; dot_green = true; } } else { // turn LED off: digitalWrite(ledPinGreen, LOW); lightOnGreen = false; dot_green = false; } // reset to current time lastMesssageTime = millis(); } } // END LOOP void onTelegraphTapeChange() { // Do something } void onDotGreenChange() { // Do something } void onDashYellowChange() { // Do something } void onSpaceBlueChange() { // Do something }
Video:
Future Updates:
- V2 – Improve current interface
- Reduce online LED on/off display lag.
- Find or build a better text display widget.
- V3 – Convert morse code signals into text words.
- Store “dots” and “dashes” into an array.
- When the user presses the “space” button convert stored data into text and then display said text.
- Clear array in preparation for next set of “dots” and “dashes”.
- V4 – Use text to speech api to convert text words into audio.
- V5 – Use sign language api to convert text words into hand sign images.